How about a maths challenge this friday!
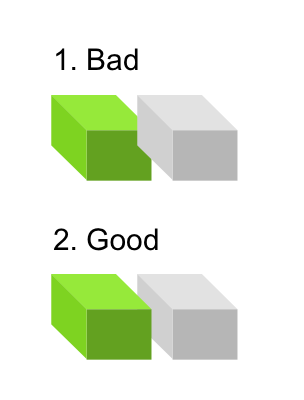
How would I go about z ordering these boxes? Currently I'm doing it by y position only, which works well for flatter objects, but not for chunky ones like above.
I have these parameters to play with for each object:
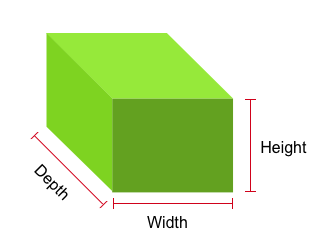
I'm trying to avoid recursively looping through all sprites, because I have a lot of them. That's why the y position solution was so good, until I started adding deep objects.
Tricky!
Comments
order according to the bottom right coord, call this (x,y).
and the order should be the following:
B needs to be rendered earlier than A if and only if (x_A smaller than x_B) OR (y_A is higher than y_B).
so basically if they are in a grid then start with the bottom right, go through e.g. the bottom row and then continue with the next row above it, again going from the right to the left. etc.
Fragmenter - animated loop machine and IKONOMIKON - the memory game
@keszegh, that could work. I would have to compare each game object with all other game objects each frame, though, which isn't really an option because I have to many of them. I could reduce the frequency I check it I suppose, but I was hoping for a one-variable silver bullet.
It won't look like your pictures though, but I am pretty sure we can craft a projection matrix that will. After all the transform should be something like (X,Y,Z) -> (X-Z*f,Y-Z*f,Z), with f being some depth factor of your choice, sqrt(2) basically.
Upcoming Viewport enhencements will allow that.
and irrespective to how the 'smaller' is defined, ordering takes nlogn time for n objects or adding an object into an order is logn time per object. these methods are completely oblivious to the definition of 'smaller' so you can just make a comparison function that says e.g. that
[EDIT]this comparison function is not correct[END of EDIT]
for sorting a table t according to this order you can use the lua function
Fragmenter - animated loop machine and IKONOMIKON - the memory game
Fragmenter - animated loop machine and IKONOMIKON - the memory game
for example if the bottomright corners are positioned as in
__x
x__
of two such objects, then it still depends on their height which one has to be rendered before the other.
however, if you get the comparison function right, then the rest applies.
(also you never said how the 'plane' of the elements is positioned, parallel to the screen or going into z coord (like an isometric tile-map) - i guess parallel to the screen, at least i thought it that way)
Likes: totebo
Fragmenter - animated loop machine and IKONOMIKON - the memory game
Output:
Code:
Likes: keszegh, totebo, vitalitymobile, antix
http://giderosmobile.com/guide
(3D graphics section)
For orthographic display you want to set the frustum like this
application:configureFrustum(0,200)
for example, where 200 is the distance to the near and far cut planes in pixels (basically make this big enough to encompass your 3D scene)
And, yes, it will be very CPU efficient. OpenGL will do all the z-ordering for you, in fact it will all happen on the GPU which is highly optimised for this task.
Likes: antix, simwhi, SinisterSoft
https://github.com/gideros/gideros
https://www.youtube.com/c/JohnBlackburn1975
I think a small class that can render a simple 3D mesh with textures would be really cool. It could be used for games where you are using 2D but want to have 3D items in inventory screens and stuff.
https://github.com/gideros/gideros
https://www.youtube.com/c/JohnBlackburn1975
@antix, x axis won't work regrettably. See illustration below. I'm trying to get a combination of x and y going, but so far no luck.
Likes: john26
Fragmenter - animated loop machine and IKONOMIKON - the memory game
Here is a very interesting article about "Drawing isometric boxes in the correct order" that you might find useful.. http://shaunlebron.github.io/IsometricBlocks/. Actually I bookmarked it because it's quite fantastic
@john26 I agree with simwhi, don't cut anything. If you feel its too long you could put in one of those note things where you can say "skip to 10:52" if you already know how to start a new project or something.
I seem to be stuck with Lua's sort function currently. Here is an illustration of the theoretical solution I've come up with to solve the problem:
This is an example of the table I need to sort (z_objects).
I also tried the isometric link above, which doesn't work, I think, because my objects are skewed, and not straight up isometric.
Taking a break from this, but do let us know if you feel you have an idea that can help.
In the example there are 6 boxes that move about the screen. Bump collision bounces them off eachother and the screen edges. They get sorted and drawn in the correct order in the sortThings() function of the Game class.
I hope it's of some use to you @totebo and maybe others too
Likes: totebo
Likes: antix
This better example just uses a filter to sort the things table which makes things a lot tidier. It also does away with collision stuff and just ensures that no things overlap when they are created.
Likes: totebo, pie, john26